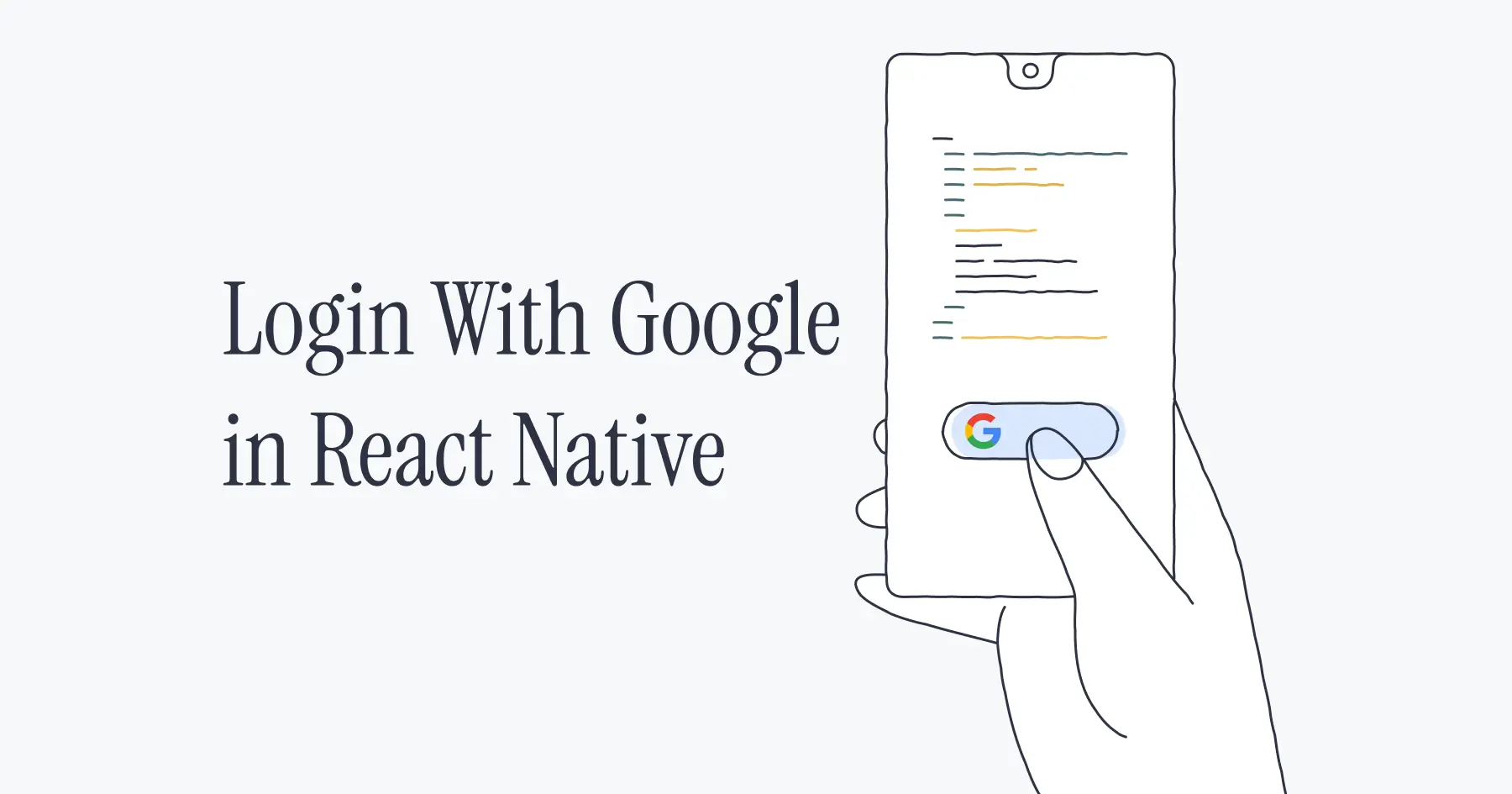
Implement login with Google in React Native
A tutorial on how to enable Google Sign-In in React Native. It covers setup, UI, user validation, and sessions for a secure and user-friendly authentication
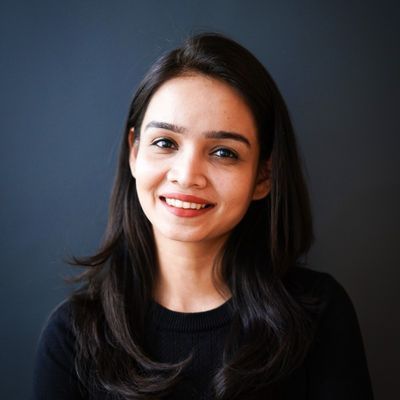
Yogini Bende
Feb 20, 2024 • 7 min read
For almost all apps you use, you have different ways to log in. You can use an email-password combination or SSO (Single Sign-On) options like Google, Apple, Github, etc. The latter option does not need us to remember the password for every app and that is why it is the most popular choice among users.
Recently, we started building a mobile app for Peerlist and added Login with Google functionality in it. While doing this, I also encountered some problems. Hence in this article today, I will walk you through the steps to enable Google Sign-In in your React Native application along with some common errors you may get and how to fix them!
Let's dive in!
Table of Contents
- Create a Google Developer Console Project
- Set Up Your React Native Project
- Install React Native Google Sign-In Package
- Configure Google Sign-In
- Create a Google Sign-In Button
- Handle Google Sign-In
- Validate the Google User
- Implement User Sessions
- Logging Out
- Possible problem: developer_error
- Bonus: React Native Jobs
- Final Thoughts
1. Create a Google Developer Console Project
Before you can implement Google Sign-In in your React Native app, you need to set up a project in the Google Developer Console:
- Go to the Google Developer Console.
- Create a new project or select an existing one.
- In the project dashboard, navigate to "APIs & Services" > "Credentials."
- Click on "Create credentials" and select "OAuth client ID."
- Choose "Web application" as the application type.
- You will also need to create one “Android” and one “iOS” application in a similar way.
- If you are stuck at finding the SHA-1 key for creating an Android Client Id, read the 10th point in the same article.
- You will need client IDs from all these 3 applications.
2. Set Up Your React Native Project
If you don't have a React Native project set up, you can create one using the following command:
npx react-native init MyGoogleSignInApp
Navigate to your project directory:
cd MyGoogleSignInApp
3. Install the React Native Google Sign-In Package
To use Google Sign-In in your React Native app, you need the @react-native-google-signin/google-signin
package. You can find this package here.
Install it by running:
npm i @react-native-google-signin/google-signin
4. Configure Google Sign-In
In your React Native app, configure Google Sign-In with your OAuth client ID. This step is essential for enabling Google Sign-In.
Open your project's main JavaScript file (e.g. index.js
) and add the following code:
import { GoogleSignin } from '@react-native-google-signin/google-signin';
import {
GOOGLE_WEB_CLIENT_ID,
GOOGLE_ANDROID_CLIENT_ID,
GOOGLE_IOS_CLIENT_ID,
} from '@env';
GoogleSignin.configure({
webClientId: GOOGLE_WEB_CLIENT_ID,
androidClientId: GOOGLE_ANDROID_CLIENT_ID,
iosClientId: GOOGLE_IOS_CLIENT_ID,
scopes: ['profile', 'email'],
});
Since this tutorial is only for Google Sign-in, I have asked to add in index.js
but if you have an existing codebase you can create a separate service for Google and add the code there.
Saving client-ids in the .env
file is always a safe choice and the above code assumes that you have a .env
file already created and all our client-ids from step 1 will be added in this.
Considering your use case you can increase the scope in the above config but for this tutorial, I have kept the scope minimal.
5. Create a Google Sign-In Button
To provide users with the option to sign in with Google, we will need a button in your React Native UI. For this, I created a simple button component using Pressable
from React Native. You can customize this button or component suitable to your app’s UI and hence I am skipping the details of styling etc.
import { useState } from 'react';
import { View, Pressable } from 'react-native';
import { GoogleSignin } from '@react-native-google-signin/google-signin';
import {
GOOGLE_WEB_CLIENT_ID,
GOOGLE_ANDROID_CLIENT_ID,
GOOGLE_IOS_CLIENT_ID,
} from '@env';
GoogleSignin.configure({
webClientId: GOOGLE_WEB_CLIENT_ID,
androidClientId: GOOGLE_ANDROID_CLIENT_ID,
iosClientId: GOOGLE_IOS_CLIENT_ID,
scopes: ['profile', 'email'],
});
const GoogleLogin = async () => {
await GoogleSignin.hasPlayServices();
const userInfo = await GoogleSignin.signIn();
return userInfo;
};
export default function App() {
const [error, setError] = useState('');
const [loading, setLoading] = useState(false);
const handleGoogleLogin = async () => {
setLoading(true);
try {
const response = await GoogleLogin();
const { idToken, user } = response;
if (idToken) {
const resp = await authAPI.validateToken({
token: idToken,
email: user.email,
});
await handlePostLoginData(resp.data);
}
} catch (apiError) {
setError(
apiError?.response?.data?.error?.message || 'Something went wrong'
);
} finally {
setLoading(false);
}
};
return (
<View>
<Pressable onPress={handleGoogleLogin}>Continue with Google</Pressable>
</View>
);
}
6. Handle Google Sign-In
In the handleGoogleLogin
function, we are performing 2 steps. Login and validate the token.
In step 1 We will attempt to sign in with Google when the button is pressed. This function performs the following steps:
- Check if the user's device has Google Play Services installed.
- Initiates the Google Sign-In process.
- If successful, it retrieves user information.
In step 2 We will send the token we received from Google and send it to our backend for validation.
7. Validate the Google User
From handleGoogleLogin
step 1, we get the idtoken
and email
from the user. Usually, we need to send that token to our backend to save it to db. But before saving, we should check the authenticity of the token as it is coming from the network
Your Next.js or other backend APIs should handle this part. Your API should make a request to the Google API along with this token something like this -
await axios({
method: 'get',
url: `https://www.googleapis.com/oauth2/v3/tokeninfo?id_token=${token}`,
withCredentials: true,
});
I will not dive into more details of this implementation as you can read more about this in Google’s documentation. Ensure you securely validate the user with Google and create or authenticate the user.
8. Implement User Sessions
To maintain user sessions in your React Native app, you can use techniques like JWT (JSON Web Tokens) or secure sessions. These mechanisms help keep track of user logins and provide a seamless user experience. In the Peerlist app implementation, we attached the session to the user’s profile hence you can do something similar.
9. Logging Out
To perform the logout feature with Google you will need to use GoogleSignin.signOut()
to allow users to log out from their Google account.
async function handleGoogleLogout() {
try {
await GoogleSignin.signOut();
// Perform additional cleanup and logout operations.
} catch (error) {
console.log('Google Sign-Out Error: ', error);
}
}
10. Possible problem: developer_error
This particular issue might occur to you while you are building Login with Google functionality for your app. Possible reasons for this error are
-
The SHA-1 key is mismatched.
- Make sure the SHA-1 you are using for creating Google’s oAuth Client and the one you used in your Android Keystore should be the same.
- To get the SHA-1 key for Android, go to the Android folder and run this command:
keytool -list -v -keystore app/debug.keystore
- It might ask you for the password, and in most cases it
android
-
Different Client-IDs
- One of the reasons for this error is a mismatch in the client IDs. Hence check your client IDs for your App and your backend twice!
- For Android, make sure the Client IDs you are using are present in
google-services.json
file. - For iOS, you will need to add iOS Client ID in
Info.plist
file inside<array>
something like this. (Do this only if it is not already added)
<dict> <key>CFBundleTypeRole</key> <string>Editor</string> <key>CFBundleURLSchemes</key> <array> <string>com.googleusercontent.apps.--your--client--id--</string> </array> </dict>
-
Different Package Name: While creating Client IDs make sure, the bundle and package names present in the
app.json
file are exactly same while creating iOS and Android Client IDs. -
You can also check this documentation. It has some detailed steps to fix this error.
Bonus: React Native Jobs
If you are looking for a job in React Native, check out the these jobs on Peerlist. You can also post your job openings for free!
Final Thoughts
Implementing Google Sign-In in your React Native app provides users with a convenient and secure way to authenticate. It's crucial to handle user information and sessions responsibly, ensuring the security and privacy of your users.
I tried to add the steps as detailed as possible. I am pretty sure that, with this tutorial, you can enable Google Sign-In in your React Native app and seamlessly integrate it with your backend APIs.
Hope you are now able to perform Login with Google in your React Native App! If yes, do share this article with your network. Cheers 🥂